Java is one of the most popular programming languages. One of the primary reasons for this popularity is mainly because of the Object-Oriented Programming approach.
Object-oriented programming (OOPs) concepts are among the most fundamental programming paradigms. It is not just an interview topic. It’s one of the most practical approaches towards building scalable apps.
In this detailed blog, we will look into everything you need to know for working with OOP in Java. Without further ado, let’s get started.
Understanding Object-Oriented Programming (OOP)
As the name indicates, the term Object-oriented programming (OOP) refers to a programming paradigm that is based on the concept of objects, which are collections of data and functions that work together to perform certain tasks.
The main idea behind OOP is to break down a complex problem into smaller logical units called classes and objects. A class is simply a blueprint for creating objects and where objects are instances of a class. This approach helps developers like you to break down complex programs into smaller and reusable code.
Object-oriented programming has four main principles. We will go through each of them in detail but before that, let’s first understand an important concept in Java, access modifiers.
Access Modifiers in Java
While talking about OOPs, access modifiers are an important aspect to consider for controlling the visibility and accessibility of class members.
Java has mainly four types of access modifiers:
Private
The private modifier restricts access to class members. Class members are the variables, methods, constructors etc. It is the most restrictive access level and is primarily used to encapsulate data by hiding sensitive data and preventing direct manipulation of class members from other classes.
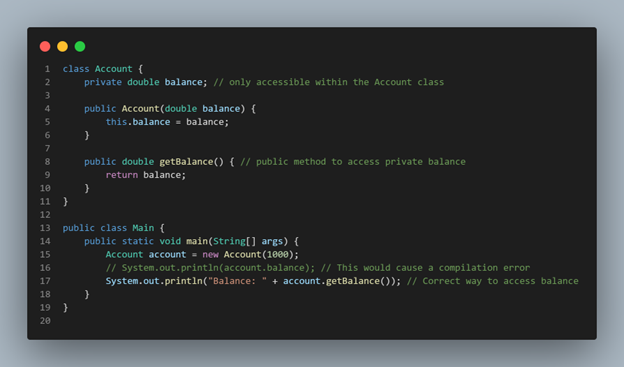
Here the balance field is a private variable and can be accessed with the public getBalance() method, this way the data is encapsulated.
Public
The public modifier is another modifier that allows you to access a class or its members from anywhere in the program. They are not restricted by class, package or inheritance hierarchy.
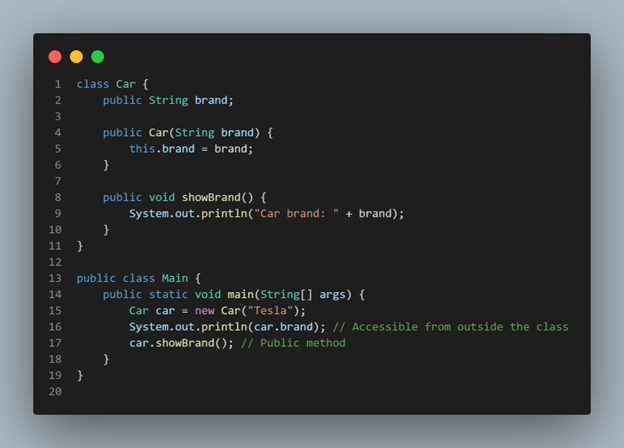
Protected
The protected modifier is another useful modifier that allows you to access the class members within the same package and by subclasses.
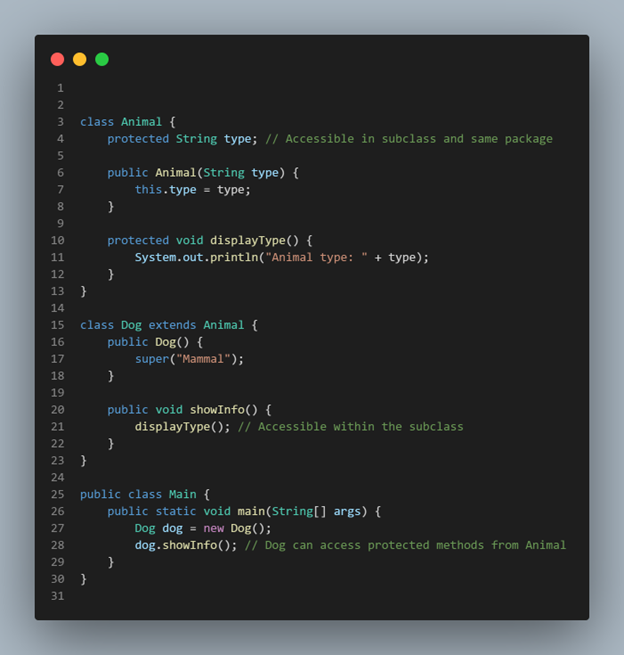
Default (Package-private)
It is the default modifier if you haven’t explicitly mentioned the modifier. If it is using a default modifier, it means that the class members are accessible only within the same package, but not from outside the package.
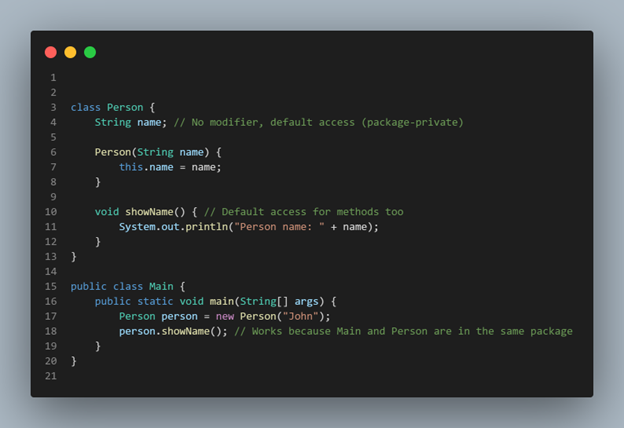
Four Pillars of Object-Oriented Programming
Now that we know about access modifiers let’s discuss the 4 pillars of Object-oriented programming: Encapsulation, Inheritance, Polymorphism and Abstraction
Data Encapsulation
Encapsulation refers to the idea of wrapping the data and functionality together inside a class. It hides the internal workings of the object from the outside world, providing access through public methods only.
Here is an example:
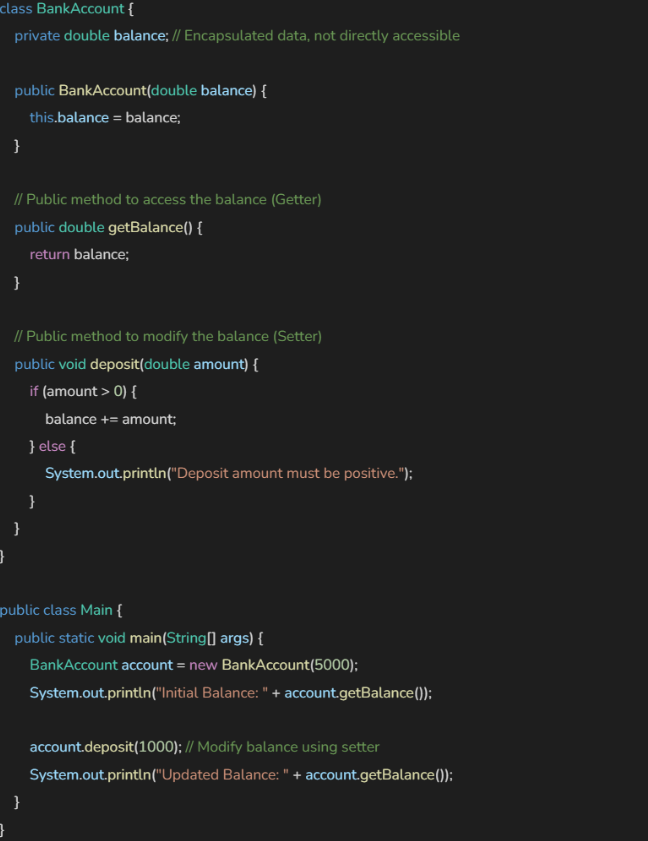
Here in the code, you can see that we have a private variable balance and it cannot be accessed directly. You have to invoke the getBalance() which is a getter method, that allows safe access to the balance.
The setter method deposit() lets you modify the balance, ensuring that only positive values can be deposited. This encapsulation protects the balance field from unwanted or incorrect modifications while allowing controlled access through public methods.
Key Benefits of Encapsulation
- Encapsulation protects the integrity of the object by preventing unintended changes to internal data.
- Since the data can only be accessed and modified by setters and getters, it promotes modularity and maintainability.
Inheritance
Inheritance is another important pillar of Object-Oriented Programming. Inheritance is the process where one child class can inherit all the properties of another class which is the parent class. This way, you can create new classes based on the existing ones and modify the new classes as you need.
In Java, there are mainly four types of inheritance:
- Single Inheritance: This occurs when a class (child class) inherits from only one superclass (parent class).
- Multilevel Inheritance: Multilevel inheritance involves a hierarchy where a subclass inherits from a superclass, which in turn inherits from another superclass.
- Hierarchical Inheritance: Here, multiple subclasses inherit from a single superclass.
- Multiple Inheritance (through Interfaces): Java does not support multiple inheritance directly. A class cannot inherit from multiple classes directly. This is to avoid ambiguity. Although your class can implement multiple interfaces. This allows a form of multiple inheritance.
Here is a simple example to demonstrate inheritance.
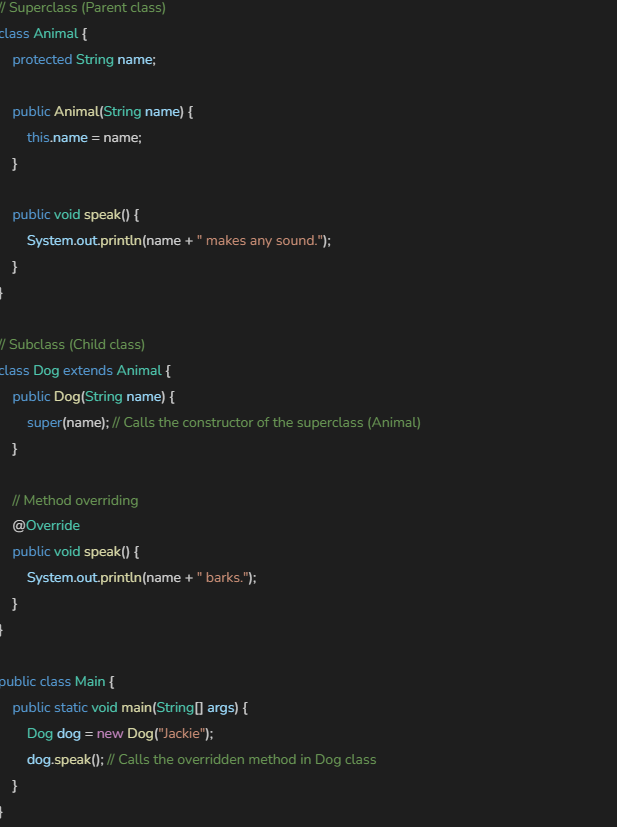
In the above example, the Dog class inherits from the base class Animal. When we call the speak() method on the dog object, it would have invoked the speak() method in the Animal class, Although in this case, we have overridden it by creating a speak() method to provide a specific implementation for dogs.
Polymorphism
Polymorphism is the ability of different objects to respond to the same method call in different ways. In OOPs, polymorphism allows you to create objects that share a common interface, but they have different implementations. Polymorphism can make your code more flexible and allows you to write code that is more easily maintainable and extensible.
For instance, you could design an Adder class with methods named add that perform different types of addition, such as adding two integers, adding three integers, adding two doubles, and concatenating two strings.
Abstraction
Abstraction is another pillar of Object-oriented programming. It is the process of hiding the details of the object implementation and showing only the necessary information to the user. In Java, you can implement abstraction by using interfaces or abstract classes.
An abstract class can have both concrete and abstract methods, while an interface only declares methods without implementation. Abstract classes are restricted from creating objects from it. To access the abstract class, it must be inherited from another class
Here is an example to show abstraction works in Java
In the above example, you can see that users can interact with the cars through a minimal interface and we hide all the implementation details from the user. This way our code is more readable and manageable.
Key Benefits of Abstraction:
- Simplifies complex systems by showing only the necessary details to the user.
- Promotes modular design by separating the abstract interface from the implementation.
Conclusion
Thanks for checking this blog. In this detailed blog, we have looked into Object-oriented programming in Java. We have seen various examples of the implementation of the four pillars of OOPs.
Having a good understanding of these OOP concepts is essential if you want to use them well and write industry-level code. By following this you will not only improve your coding skills but also build applications that can adapt to change and grow over time.
Keep learning!
Read more such articles on Frontend Engineering here.